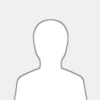 |
Hi!
The rule states:
Quote:Expressions with type enum or enum class shall not be used as operands to built-in and overloaded operators other than the subscript operator [ ], the assignment operator =, the equality operators == and ! =, the unary & operator, and the relational operators <, <=, >, >=.
I can understand the rule insofar as to avoid confusion that is caused by using the underlying type arithmetically. However, this also excludes the ternary conditional operator, and thus, this IMHO perfectly fine code:
Code: void foo()
{
enum class Thing { One, TheOther };
bool which_one = g();
const Thing selected_thing = which_one ? Thing::One : Thing::TheOther; // Non-compliant with A4-5-1
}
Please note that turning this into an "if" will add another statement and preclude "selected_thing" from being const. Depending on the C++ version, this also precludes the surrounding function from being constexpr (because of the extra statement). In my opinion, the demonstrated usage should be allowed in general.
Is it an oversight to disallow enum values in these cases, or is there a specific reason? If there is a reason, I would urge you to overthink your stance on this w.r.t. the upcoming MISRA C++.
Edit: Also, this code violates A5-16-1, because it uses the ternary conditional operator as a subexpression of the assignment expression. I guess this is an oversight in A5-16-1, which should have an exception for assignment expressions.
Thanks!
|
|
|