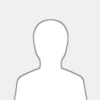 |
Hi,
Rule 7.0.2 bans any type of conversion to bool (implicit or explicit) anywhere in the code (with some exceptions). The rationale says:
"However, this interpretation may not be appropriate for APIs, such as POSIX, that do not use Boolean return values."
This sounds strange, why should modern C++17 code (that may not use C-style POSIX functions at all) be limited by this?
Could you show an example code that highlights how this is a problem and leads to unexpected behavior? For example, how is this code unsafe/can lead to unexpected results?
Code: bool b1 = static_cast< bool >( 4 ); // Non-compliant
Conversion from fundamental types to bool is well-defined behavior as per [conv.bool]:
"A prvalue of arithmetic, unscoped enumeration, pointer, or pointer to member type can be converted to a prvalue of type bool. A zero value, null pointer value, or null member pointer value is converted to false; any other value is converted to true."
Thanks!
|